728x90
반응형
-
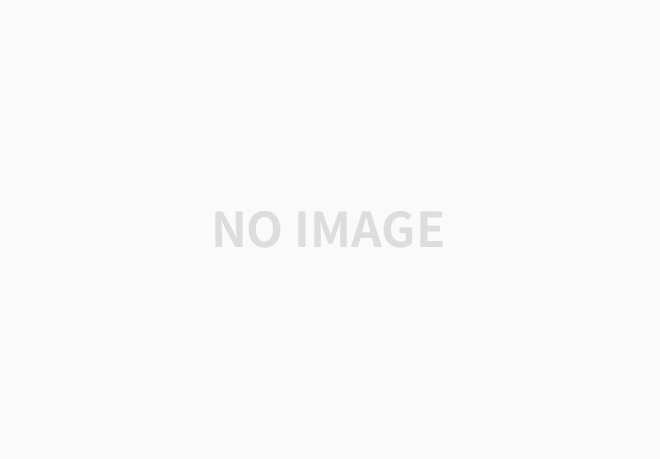
-
안녕하세요? 오늘은 카카오 알림톡 구현 방법에 대해서 알아볻보록 하겠습니다.
카카오 알림톡 구현 방법에 대해서는 여러가지 방법이 있지만, 이번 포스팅에서는 NHN Cloud를 이용하여, NHN Notification의 KakaoTalk Bizmessage 서비스를 사용한 방식에 대해서 알아보도록 하겠습니다.
728x90
※ 해당 카카오 알림톡은 1. 조직 등록 > 2. 프로젝트 등록 > 3. 서비스 선택 > Notification > KakaoTalk Bizmessage > 발신 프로필이 등록작업이 선행되어야 합니다.
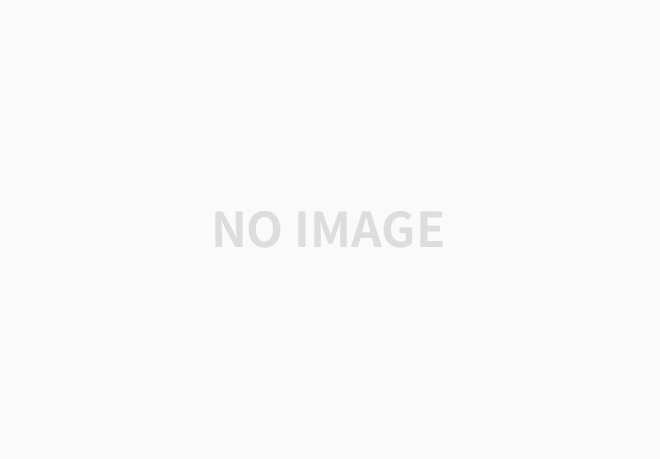
해당 작업이 완료되고 나면, 서비스 이용에 필요한 Key들인 App key와 Secret Key, Sender Key를 발급받을 수 있습니다.
1. 알림톡 템플릿 등록
NHN Cloud Console의 프로젝트 하위의 Notification > KakaoTalk Bizmessage > 알림톡 > 템플릿 관리 메뉴로 진입합니다.
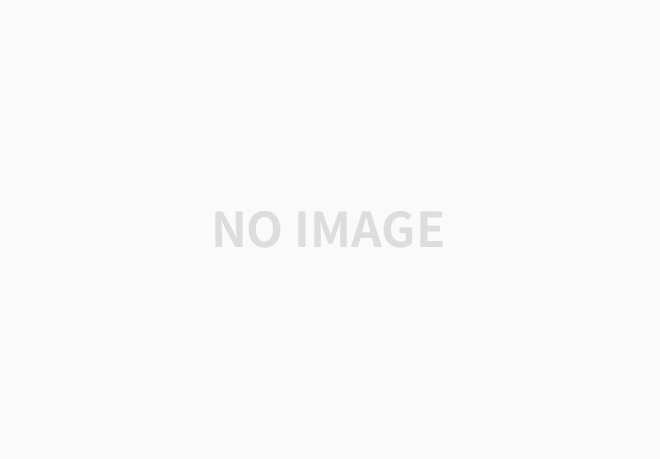
템플릿 등록 버튼 클릭 시, 아래와 같이 템플릿 등록 팝업이 나타나게 됩니다.
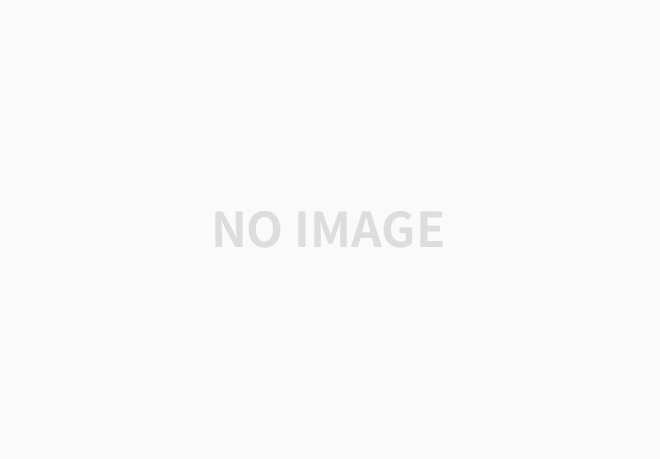
사용할 용도에 맞게 템플릿을 구성 후, 저장 합니다.
2. Java 구현
* 실제 카카오 알림톡 전송부
package kakao;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Pattern;
import org.apache.log4j.Logger;
import org.json.simple.JSONArray;
import org.json.simple.JSONObject;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
import atp.AtpUtil;
import coremethod.util.CommonUtil;
import lombok.RequiredArgsConstructor;
@RequiredArgsConstructor
@Service
public class KakaoService {
private static final Logger log = Logger.getLogger(AtpUtil.class.getName());
// 카카오 알림톡 API 도메인 및 엔드포인트
private static final String ALIMTALK_API_DOMAIN = "https://api-alimtalk.cloud.toast.com";
private static final String ALIMTALK_API_URL = "/alimtalk/v2.3/appkeys";
// 비즈니스 계정 관련 키값 (환경 변수 또는 설정 파일에서 관리하는 것이 바람직함)
private static final String BIZ_APP_KEY = "AAA";
private static final String BIZ_SECRET_KEY = "BBB";
private static final String BIZ_SENDER_KEY = "CCC";
/**
* 카카오 알림톡 메시지를 전송한다.
*
* @param params 메시지 수신자 목록 및 템플릿 정보
* @return API 응답 데이터 (성공/실패 여부 포함)
* @throws Exception 예외 발생 시 로깅 후 예외 전파
*/
public Map<String, Object> sendKakaoAlimTalk(List<Map<String, Object>> params) throws Exception {
Map<String, Object> apiParamMap = new HashMap<String, Object>();
if (params != null && !params.isEmpty() && params.size() > 0) {
// 수신자 목록을 JSON 배열로 변환 후, 단건으로 전송 : 추후 로그 추적용으로 단건 처리
for (Map<String, Object> param : params) {
try {
// 모든 파라미터 길이 합산
Map<String, String> templateParams = (Map<String, String>) param.get("templateParameter");
int totalLength = templateParams.values().stream().mapToInt(String::length).sum();
if (totalLength > 750) {
log.error("카카오 알림톡 템플릿 바디 생성 중 오류 발생 (1000자 제한 초과) : ");
} else {
// 수신자가 여려명일 경우
if ((boolean) param.get("recipientMultiFlag")) {
// 수신자 번호 목록 가져오기
List<String> recipientNoList = (List<String>) param.get("recipientNoList");
// 수신자 번호가 존재하는지 확인
if (recipientNoList != null && !recipientNoList.isEmpty()) {
// 수신자 목록을 처리하는 로직 추가
for (String recipientNo : recipientNoList) {
Map<String, Object> tempParam = new HashMap<String, Object>();
tempParam.put("templateCode", param.get("templateCode"));
tempParam.put("recipientNo", recipientNo);
tempParam.put("templateParameter", param.get("templateParameter"));
JSONObject recipientJson = this.getTemplateBody(tempParam);
if (recipientJson != null && !"".equals(recipientJson)) {
// API 호출
apiParamMap = this.callRestApi(String.valueOf(param.get("templateCode")), recipientJson);
} else {
log.error("카카오 알림톡 전달 가공 데이터 미존재");
apiParamMap.put("code", 500);
apiParamMap.put("message", "API 호출 중 오류 발생");
}
}
} else {
log.error("수신자 번호 목록이 비어 있습니다.");
apiParamMap.put("code", 500);
apiParamMap.put("message", "수신자 번호 목록이 비어 있습니다.");
}
// 수신자가 한명일 경우
} else {
// 휴대폰 형식 및 길이 체크
JSONObject recipientJson = this.getTemplateBody(param);
if (recipientJson != null && !"".equals(recipientJson)) {
// API 호출
apiParamMap = this.callRestApi(String.valueOf(param.get("templateCode")), recipientJson);
} else {
log.error("카카오 알림톡 전달 가공 데이터 미존재");
apiParamMap.put("code", 500);
apiParamMap.put("message", "API 호출 중 오류 발생");
}
}
}
} catch (Exception e) {
log.error("카카오 알림톡 템플릿 바디 생성 중 오류 발생 : ", e);
}
}
} else {
//throw new IllegalArgumentException("전송할 메시지 데이터가 비어 있습니다.");
log.error("카카오 알림톡 전달 원본 데이터 미존재");
apiParamMap.put("code", 500);
apiParamMap.put("message", "API 호출 중 오류 발생");
}
return apiParamMap;
}
/**
* REST API를 호출하여 알림톡을 전송한다.
*
* @param templateCode 템플릿 코드
* @param recipientJson 수신자 목록 JSON
* @return API 응답 데이터
*/
private Map<String, Object> callRestApi(String templateCode, JSONObject recipientJson) {
String bizApiUrl = ALIMTALK_API_DOMAIN + ALIMTALK_API_URL + "/" + BIZ_APP_KEY + "/messages";
// HTTP 요청을 위한 RestTemplate 생성
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
headers.set("X-Secret-Key", BIZ_SECRET_KEY);
headers.set("Content-Type", "application/json; charset=UTF-8"); // UTF-8 인코딩 설정
// 요청 JSON 데이터 생성
JSONObject paramJson = new JSONObject();
paramJson.put("senderKey", BIZ_SENDER_KEY);
paramJson.put("templateCode", templateCode);
ArrayList<JSONObject> arrayJson = new ArrayList<JSONObject>();
arrayJson.add(recipientJson);
paramJson.put("recipientList", arrayJson);
// 응답 데이터 저장용 맵
Map<String, Object> resultMap = new HashMap<>();
try {
// HTTP 요청 생성 및 전송
HttpEntity<String> requestEntity = new HttpEntity<>(paramJson.toString(), headers);
ResponseEntity<String> responseEntity = restTemplate.postForEntity(bizApiUrl, requestEntity, String.class);
// 응답 데이터 추출
String responseBody = responseEntity.getBody();
resultMap.put("code", responseEntity.getStatusCodeValue());
resultMap.put("message", responseEntity.getStatusCode().getReasonPhrase());
// 응답이 성공인 경우 JSON 데이터 파싱
if (responseEntity.getStatusCode() == HttpStatus.OK && responseBody != null) {
JsonParser parser = new JsonParser();
JsonObject responseData = parser.parse(responseBody).getAsJsonObject();
resultMap.put("responseData", responseData);
} else {
log.error("카카오 알림톡 API 호출 중 오류 발생");
resultMap.put("code", 500);
resultMap.put("message", "API 호출 중 오류 발생");
}
} catch (Exception e) {
log.error("카카오 알림톡 API 호출 중 오류 발생", e);
resultMap.put("code", 500);
resultMap.put("message", "API 호출 중 오류 발생");
} finally {
// 필요하다면 정리 작업 수행 (예: 특정 변수 초기화 등)
}
return resultMap;
}
/**
* 템플릿 메시지 본문을 생성한다.
*
* @param param 수신자 정보 및 템플릿 치환 파라미터
* @return 메시지 본문 JSON 객체
*/
private JSONObject getTemplateBody(Map<String, Object> param) {
JSONObject recipientJson = new JSONObject();
// 필수 데이터 검증
if (!param.containsKey("templateCode") || !param.containsKey("recipientNo") || !param.containsKey("templateParameter")) {
log.error("템플릿 메세지 생성 파라메터 미존재");
recipientJson = null;
} else {
String recipientNo = String.valueOf(param.get("recipientNo"));
recipientNo = recipientNo.replaceAll("-", "");
// 휴대폰 번호 형식 및 길이 검증 (숫자만 허용, 10~11자리)
if (!isValidPhoneNumber(recipientNo)) {
log.error("유효하지 않은 휴대폰 번호 형식 : " + recipientNo);
return null;
}
recipientJson.put("recipientNo", recipientNo); // 수신자 번호
// templateParameter 맵을 JSONObject로 변환하여 전달
Map<String, String> templateParams = (Map<String, String>) param.get("templateParameter");
JSONObject templateParameterJson = new JSONObject();
// 템플릿 파라미터 값이 Map 형태로 들어오므로 이를 JSON으로 변환
for (Map.Entry<String, String> entry : templateParams.entrySet()) {
templateParameterJson.put(entry.getKey(), entry.getValue());
}
recipientJson.put("templateParameter", templateParameterJson); // 치환될 파라미터
}
return recipientJson;
}
/**
* 휴대폰 번호 검증 메서드 (숫자만 포함, 10~11자리)
*
* @param phoneNumber 검증할 휴대폰 번호
* @return 유효 여부 (true: 유효, false: 유효하지 않음)
*/
private boolean isValidPhoneNumber(String phoneNumber) {
return phoneNumber != null && Pattern.matches("^\\d{10,11}$", phoneNumber);
}
/**
* 동적으로 value 키를 만들어서 템플릿 파라미터를 생성하는 메서드
*
* @param param 템플릿 파라미터
* @return Map<String, String>
*/
public static Map<String, String> createTemplateParameters(List<String> values) {
Map<String, String> templateParameter = new HashMap<>();
// 값이 들어오는 순서대로 자동으로 value1, value2, value3 ... 생성
for (int i = 0; i < values.size(); i++) {
String key = "value" + (i + 1); // value1, value2, value3 ...
String value = (values.get(i) == null)? "-" : values.get(i); // 해당 인덱스의 값
templateParameter.put(key, value); // map에 추가
}
return templateParameter;
}
}
* 전송부 호출용 전송 로직 예제
package kakao;
import java.util.*;
import org.apache.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class KakaoServiceCaller {
private static final Logger log = Logger.getLogger(KakaoServiceCaller.class);
@Autowired
private KakaoService kakaoService;
/**
* 단일 수신자에게 알림톡을 보내는 메서드
*
* @param recipientNo: 알림을 받을 휴대폰 번호
* @param templateCode: 사용할 템플릿 코드
* @param values: 템플릿에 들어갈 값 리스트
* @memo recipientMultiFlag를 false로 설정하여 단일 수신자로 전송
*/
public void sendSingleAlimTalk(String recipientNo, String templateCode, List<String> values) {
try {
Map<String, Object> param = new HashMap<>();
param.put("recipientNo", recipientNo);
param.put("templateCode", templateCode);
param.put("templateParameter", KakaoService.createTemplateParameters(values));
param.put("recipientMultiFlag", false);
List<Map<String, Object>> params = Collections.singletonList(param);
Map<String, Object> response = kakaoService.sendKakaoAlimTalk(params);
log.info("알림톡 전송 결과: " + response);
} catch (Exception e) {
log.error("알림톡 전송 중 오류 발생", e);
}
}
/**
* 다중 수신자에게 알림톡을 보내는 메서드
*
* @param recipientNos: 알림을 받을 휴대폰 번호 목록
* @param templateCode: 사용할 템플릿 코드
* @param values: 템플릿에 들어갈 값 리스트
* @memo recipientMultiFlag를 true로 설정하여 다중 수신자로 전송
*/
public void sendMultipleAlimTalk(List<String> recipientNos, String templateCode, List<String> values) {
try {
Map<String, Object> param = new HashMap<>();
param.put("recipientNoList", recipientNos);
param.put("templateCode", templateCode);
param.put("templateParameter", KakaoService.createTemplateParameters(values));
param.put("recipientMultiFlag", true);
List<Map<String, Object>> params = Collections.singletonList(param);
Map<String, Object> response = kakaoService.sendKakaoAlimTalk(params);
log.info("알림톡 전송 결과: " + response);
} catch (Exception e) {
log.error("알림톡 전송 중 오류 발생", e);
}
}
}
제공받은 Key들을 토대로, 전송 테스트 결과를 실시간으로 Console에서 확인이 가능합니다.
반응형
이렇게 오늘은 카카오 알림톡 서비스 구현에 대하여 알아보았습니다.
오늘도 즐거운 하루 되시길 바라겠습니다.
728x90
반응형
'프로그래밍 > Back-end' 카테고리의 다른 글
자바에서 소수점 버림 처리하는 다양한 방법 (0) | 2024.11.18 |
---|---|
자바에서 소수점 올림과 반올림 처리하는 다양한 방법 (0) | 2024.11.16 |
Java에서 정규식(Regex) 사용법과 메타문자 소개 (0) | 2024.11.15 |
자바에서 서버의 호스트명을 가져오는 방법 (0) | 2024.11.13 |
자바에서 경로 다루기: File, Request, Session을 활용한 경로 처리 방법 (0) | 2024.11.12 |
자바 AWS SNS ( Amazon Simple Notification Service ) ! 아마존 문자 서비스 구현 ! (0) | 2024.10.25 |
자바 AWS SES ( Amazon Simple Email Service ) 아마존 이메일 서비스 구현 ! (0) | 2024.10.25 |
JPA 쿼리 JPQL 벌크 연산 (0) | 2024.10.24 |